C# Extension Methods
21 November 2017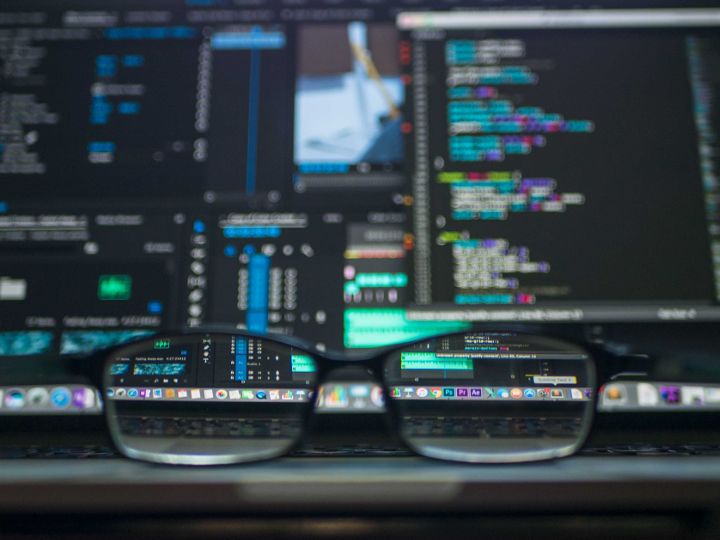
Photo: Kevin Ku - Unsplash
Code is all about readability but in itself is not very readable. One of the common statements you learn about structuring your code is to make it flow like prose. That is improved by clean naming and logical structuring and following clean code. But, languages get in the way of this by making you use operators such as !=. Ironic really.
.Net introduced Extension methods early in the history of the framework. The documents state that:
“Extension methods enable you to “add” methods to existing types without creating a new derived type, recompiling, or otherwise modifying the original type. “
You can read the full documentation here.
Extension methods are a special type of static method but you invoke them as an instance method. The following is an example of how to wrap up the IsNullEmptyOrWhiteSpace(string) String object method as an extension
public static bool IsNullEmptyOrWhiteSpace(this String s)
{
return string.IsNullOrWhiteSpace(s);
}
var testString = "This Is A Test String";
testString.IsNullEmptyOrWhiteSpace(); // false
The method has to be defined static wihtin a class that is public. It must also take a parameter matching the type it is extending as the first parameter, prefixed with the this modifier. Beyond these requirements, you can do what you want with extension methods.
One of the things that I like to do is negate methods so that you can lose the ugly ! Operator. In the case above I have a matching method defined as
public static bool IsNotNullEmptyOrWhiteSpace(this String s)
{
return !string.IsNullOrWhiteSpace(s);
}
var testString = "This Is A Test String";
testString.IsNotNullEmptyOrWhiteSpace(); // true
I find that this helps with readability and that as a project goes on you find lots of uses for Extension methods. I find you are able to actually define a DSL specific to your project which can be quite powerful. The thing I do not recommend is building business logic into your Extensions. They should be simple, type related methods that add value or improve readability.
Taking this a stage further, I have planned a library of common extensions designed to improve readability. I know that I prefer
public static bool IsNull(this object o)
{
return o == null;
}
And
public static bool IsNotNull(this object o)
{
return o != null;
}
Which gives us
var someObject = new Object();
if(someObject.IsNotNull())
{
// some code ...
}
Instead of
if(someObject != null)
{
// some code ...
}
Doesn’t that make reading the code a little easier and clearer?
If you’d like to discuss the use of Extension methods or if you have extensions you would like to contribute, message me via twitter or email.