Encoding Html Strings For SQL Storage
09 July 2019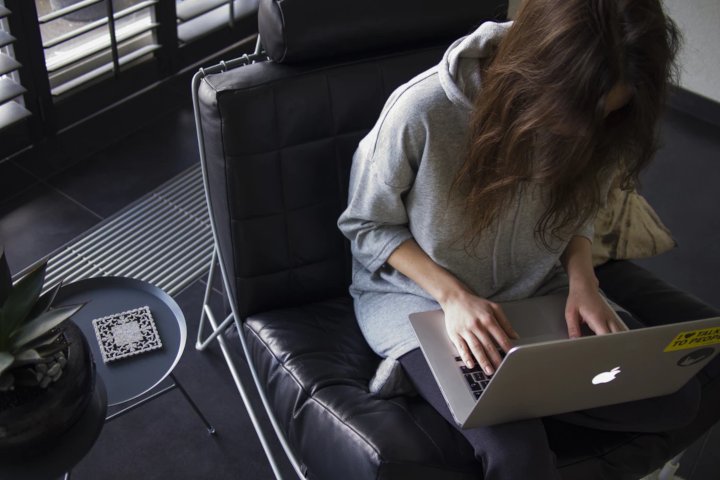
Photo: Daria Nepriakhina - Unsplash
Recently I reviewed some code that contained a problem that I hadn’t considered for a long time. Storing Html strings in a database.
The basic rule is that you should not store unencoded Html in the database for a couple of clear reasons:
- Security. If the strings stored in the database are going to be inserted to the DOM or a
<script>
tag then it should be escaped. You should be following the security guidelines (as a minimum) from the OWASP Project. Doing so will help you prevent Cross-Site Scripting (XSS). Not encoding your Html (or XML/JSON) strings facilitates XSS. - It will break your pages layouts. Users have a knack for saving data you won’t have had the chance to think about or test. If a user creates a snippet in your database that includes
</body>
then you will get a broken page when it is rendered.
Most languages and stacks have inbuilt utilities and libraries for preventing XSS. They can handle the act of encoding so you do not have to write your implementation. In C# you can use the HtpUtility classes HtmlEncode and HtmlDecode methods. The sample code below shows how this works.
using System;
using System.Web;
public class Program
{
public static void Main()
{
var htmlString = @"<!DOCTYPE html> <html> <head> <meta charset='UTF-8'> <title>Example HTML Encoding Page</title> </head> <body> <p>This Is An Example File</p></body> </html>";
Console.WriteLine("Non Encoded\n{0}", htmlString);
var encodedString = HttpUtility.HtmlEncode(htmlString);
Console.WriteLine("Encoded\n{0}", encodedString);
var decodedString = HttpUtility.HtmlDecode(encodedString);
Console.WriteLine("Dencoded\n{0}", decodedString);
}
}
In my projects, I tend to wrap the HttpUtility calls into extension methods. This means that if I have to change or add something to the implementation going forward I have one place to change.
public static class StringExtensions
{
public static string HtmlEncode(this string s)
{
return HttpUtility.HtmlEncode(s);
}
public static string HtmlDecode(this string s)
{
return HttpUtility.HtmlDecode(s);
}
}
If you have any comments or thoughts around this post then please contact me via twitter or email.