Keeping Entity Objects Anemic
10 July 2018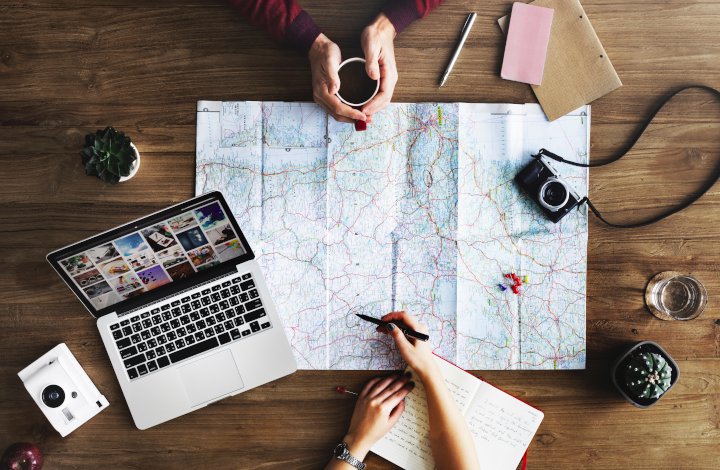
Photo: Unsplash
I’ve recently seen some Entity Framework entity classes that are doing more than they should. A good example of what I am talking about is something like the following:
public class MyEntity
{
public string Name{get; set;}
public int Age{get; set;}
public DateTime DOB{get; set;}
public void CreateMyEntityFrom(SomeOtherViewModel someOtherViewModel)
{
this.Name = someOtherViewModel.Name;
this.Age = someOtherViewModel.Age;
this.DOB = someOtherViewModel.DOB;
}
public SomeOtherViewModel CreateSomeOtherViewModelFromThis()
{
return new SomeOtherViewModel
{
Name = this.Name,
Age = this.Age,
DOB = this.DOB
};
}
}
public class SomeOtherViewModel
{
public string Name{get; set;}
public int Age{get; set;}
public DateTime DOB{get; set;}
public string AddressOne{get; set;}
public string AddressTwo{get; set;}
public string AddressThree{get; set;}
public string AddressFour{get; set;}
public string PostCode{get; set;}
}
I have put that together that code to highlight a point, hence the lack of attributes and EF scaffolding. This is a common pattern of code where you need to set ViewModel or DTO values from your entity.
The point is that EF entity classes should be anemic.
If ever a statement might get me some hate that will and I know some people disagree. But, if you are using POCO’s to represent your database records they should not encapsulate behaviour as well. Keeping your logic apart from your data makes sense. Logic and behaviour should work on and not with data. Very rarely from my experience is this not the case.
The most obvious solution to this kind of code is using an Object Mapper such as Automapper or Mapster. These libraries are designed to remove this kind of mapping code. They are also designed to handle deep object cloning (which is another post in itself).
With most of the mappers, you generally can either set up some configuration or specify the fields to map. In most projects, I set up configuration in the applications startup or initialisation stage. You would perhaps add a line such as:
AutoMapper.Mapper.CreateMap<MyEntity, SomeOtherViewModel>();
You can then call the mapping function provided by the library to populate the target object.
var somOtherViewModel = AutoMapper.Mapper.Map<SomeOtherViewModel>(myEntity);
This will map the values between the objects where the property names and types match. This approach will keep configuration code to a minimum. Most of the mapping libraries will also provide ways of specifying options when mapping values. These vary between library’s and so reading the documentation is best.
The benefit of this approach is that you can specify your mapping configurations in one place. If a change has to be made then you get to update a single code file. You don’t have to find all occurrences of object mappings to update.
There other, sometimes more beneficial, options in this area such as converters. I may revisit this area soon with more ideas.
I’m sure some views here are worthy of discussion and I’d like to hear about any other mapping library’s worthy of use. Please share them with me via twitter or email.