Readable Code Part 2 - Comments
26 March 2019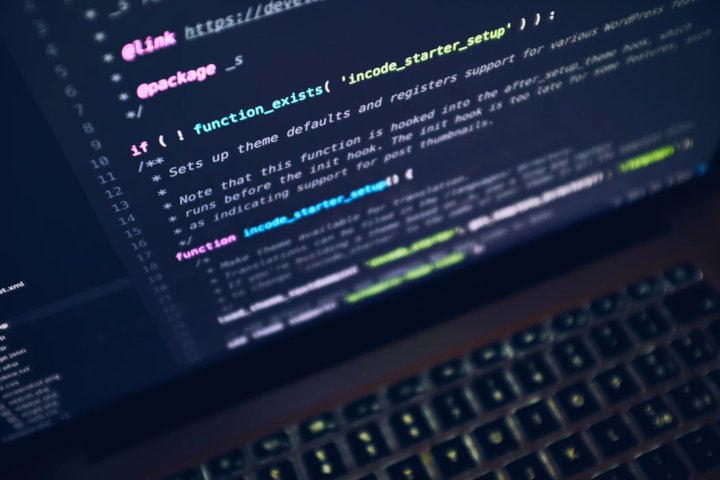
Photo: Unsplash
This post continues my series on code readability. In this post, I want to cover something that exists in all languages, comments.
All languages support single and multi-line comments. Lines marked with your languages comment characters are not executed by your compiler. Instead, they are used to provide a simple documentation mechanism within your code. While it would seem logical to use extensive comments in your code, it is almost always not the case. But, as I will cover further in the post, using comments for generating documentation can be a useful tool.
Standard code comments make reading code difficult. They extend the code height and add additional noise that makes reading the code much harder. Like prose, when you read code you want to follow the flow of the code like you would follow the text in paragraphs. With comments scattered throughout your code, you are they forced to context switch. It breaks your thinking and understanding through interruption.
Code that is extensively commented is usually always done so to support badly written code. This is what is commonly known as a code smell. The comments can usually be removed by following better naming conventions. Better structure and breaking your code up to be more readable will also reduce their need. This is something I plan to cover in the next post.
Let’s cover some examples of bad comments. First the obvious one. Never, ever repeat in the comment when it is obvious what the code is doing, such as:
// Initialise number to 99
int number = 99;
// Set isSomething to true
var isSomething = true
These comments add no value. The code’s intention is clear. Don’t do this. Also, don’t use comments to provide a running commentary on code such as
public string DoSomethingWith(Model data)
{
// Get property value from data ....
var propertyValue = data.property;
// ... apply transformation to property ...
Transform(propertyValue);
// ... return transformed property
return propertyValue;
}
Again, this adds no value but does add the noise and height I mentioned earlier. I have seen code that has this throughout the whole project and it makes reading the code really hard. Not only is it hard to read but, and we come to the main issue with excessive comments, they are likely to be out of date.
When developers write code and add extensive comments they do so selfishly. It is for their own good. They think they are doing it to be good programmers and to aid whoever comes next to work on the code. But, if that was the case then they would have written well structured and logically named code. They would not need to fall back on comments. When someone new comes to the code they may not expect or have time to maintain the comments and the code. The priority is the code and once one comment becomes out of date then the Broken Window principle kicks in. The third developer will come along and see that a comment has not been updated. They will not bother to maintain the comments around the code they have modified. As time goes on the code changes. But the comments are left to rot until someone does the decent thing and deletes them. This is the only thing left to do as a wrong comment is of no value at all.
Before I move on, there is an excellent collection of funny and inappropriate comments on Stack Overflow. None of these should be seen in any production code base no matter how amusing they are.
So when should you add comments? Easy, when it explains a non-logical snippet of code. This usually relates to business logic and the meaning is not easy to convey in code. An example could be similar to:
public DateTime GetExpiryDateTimeFrom(Model model)
{
var expiry = model.ExpiryDate;
switch(model.Type)
{
case HHType:
case NHHType:
// Power Market Prices Expire At 4pm
return new DateTime(expiry.Year, expiry.Month, expiry.Day, 16, 0, 0);
case GasType
// Gas Market Prices Expire At 6pm
return new DateTime(expiry.Year, expiry.Month, expiry.Day, 18, 0, 0);
}
}
The other main use for comments these days is for generating documentation. Python has PEP 257 and C# has XML documentation comments. Most modern languages have some form of these comments. They provide a mechanism to add comment blocks to your methods and functions. The languages then have tools or applications that use the comment blocks to create documentation. In C#’s case, the comments are extracted to an XML file on a build. There are a number of tools such as Sandcastle that can be used to build your documentation from the XML file. In fact, Visual Studio (and Code) will use the XML comments to provide Intellisense for your own code as do other editors. This can simplify the sharing of knowledge in a codebase for a team in a manner the developers already use.
So code comments can have a use when used sparingly. They should not be used to explain poorly written code, provide running commentary or berate other developers. Used wisely, like most tools, they can add value and even be used to extend your editors functionality. The main thing to remember as a good developer, if a comment is required and already exists then maintain it as if it was code. If it adds no value, remove it.
If you have any opinions around code comments or the points made here then please contact me via twitter or email.